This week I worked on finishing the rig, procedural layout in houdini, and pyro look development.
Rig
The rig has been tested and approved by our animator.
Rigging Scripts
I wrote some quick python scripts for maya to help me with this rig to automate and increase accuracy.
import maya.cmds as cmds
def joints2curve():
curve = cmds.ls(sl=1)[0]
curve_point_count = cmds.getAttr(curve + ".spans")+1
cmds.select(d=1)
prev_joint = ""
root_joint = ""
for i in range(0,curve_point_count):
joint_name = f"curve_JNT_0{i}"
joint_pos = cmds.getAttr(curve + f".ep[{i}]")[0]
joint = cmds.joint(n = joint_name, p=joint_pos)
print(joint_pos)
print(joint)
if i==0:
prev_joint = joint
root_joint = joint
continue
cmds.joint(joint, e=1, zso=1, oj = "xyz")
prev_joint = joint
cmds.joint(root_joint, e=1, oj = "xyz", secondaryAxisOrient = "xup",ch=1, zso=1)
joints2curve()
import maya.cmds as cmds
def pivot2joint():
# Select control and joint and run to snap control pivot to joint
selected = cmds.ls(sl=1)
ctrl = selected[0]
jnt = selected[1]
jnt_pos = cmds.joint(jnt, q=1, p=1)
cmds.move(jnt_pos[0],jnt_pos[1],jnt_pos[2], ctrl + ".scalePivot", ctrl + ".rotatePivot", absolute=1)
pivot2joint()
import maya.cmds as cmds
def joint2pivot():
selected = cmds.ls(sl=1)
obj = selected[0]
jnt = selected[1]
obj_rpivot = cmds.getAttr(obj + ".rotatePivot")[0]
cmds.move(obj_rpivot[0], obj_rpivot[1], obj_rpivot[2], jnt)
joint2pivot()
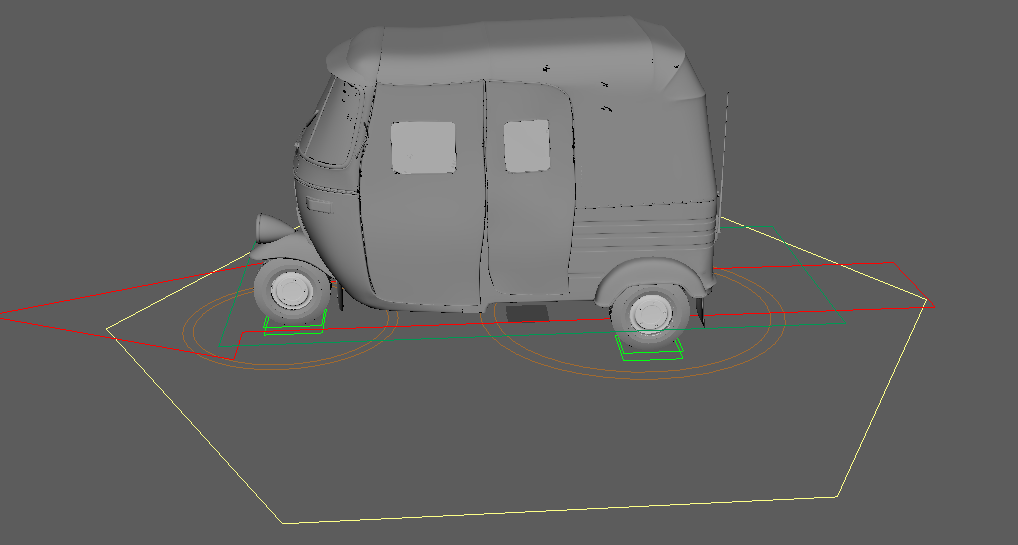