For this project I am going to experiment with the new render layers options in Maya instead of the default system. This weekend I captured live action plates for integration using a DSLR and an HDRI using a 360 camera. The photos were converted to srgb and reformatted using Nuke.

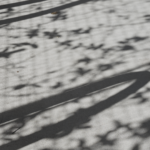
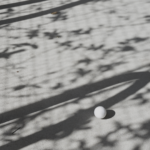
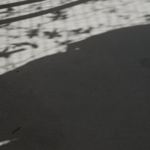
In Maya, I then camera matched the scene using the cube plate I captured, the focal length was 50mm and the camera had a slight rotation on the Z axis.
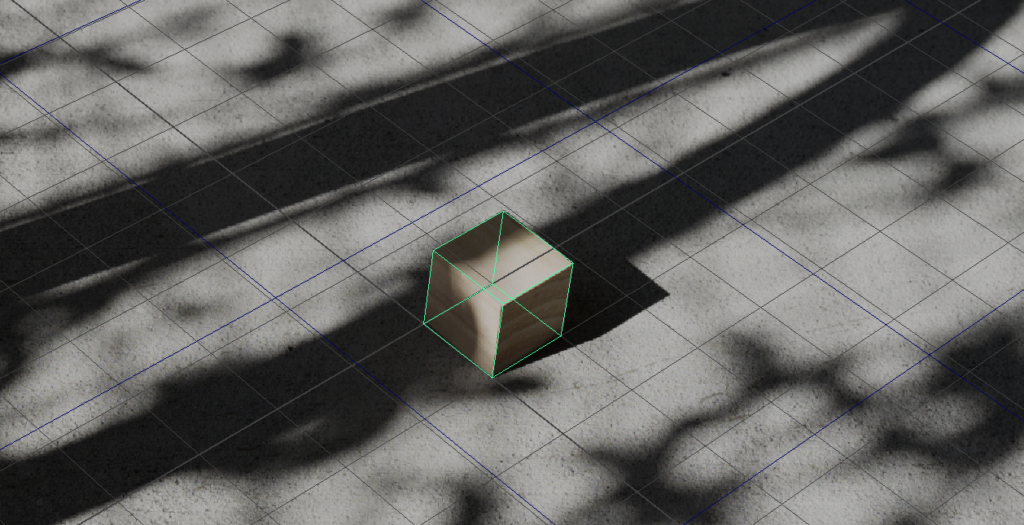
The CG element I am integrating is a baseball model from Polyhaven. I placed the model along with a ground plane into seperate render layers titled; beauty, shadow_pass, ao_ground, and ao_obj. A gray ball layer was also created for lighting reference.
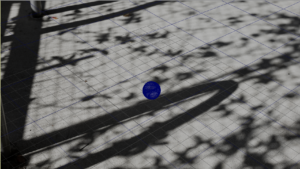
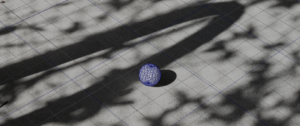
Maya doesn’t have a native tool for quickly swapping out image planes in your render layer so I created a python tool that allows for easy toggling between reference when creating a CG integration.
import maya.cmds as cmds
import os
from functools import partial
#------------------ Read Me ----------------------------------------
'''
1. Make sure project is Set
2. Place your image planes in the sourceimages folder of your project.
3. Select the camera you want to create planes for.
4. Execute script
5. Use the slider to toggle between images.
'''
#----------------------------------------------------------------------
projectDir = cmds.workspace(q = 1, rd = 1)
print(projectDir)
sourceImgDir = projectDir + 'sourceimages'
imgPlaneDir = projectDir + 'sourceimages' + '\image_planes'
if os.path.isdir(imgPlaneDir) and len(os.listdir(imgPlaneDir)) == 0:
os.rmdir(imgPlaneDir)
if not os.path.isdir(imgPlaneDir):
os.chdir(sourceImgDir)
os.makedirs('image_planes')
imgList = os.listdir(sourceImgDir)
for item in imgList[1:-1]:
os.rename(sourceImgDir + '/' + item, imgPlaneDir + '/' + item)
def getSliderValue(ctrlName):
value = cmds.intSliderGrp(ctrlName, q = 1, value = 1)
return value
selCam = cmds.ls(sl = 1)[0]
planeName = selCam + '_imgPlane'
def toggleImage(slider, *args):
value = getSliderValue(slider)
if cmds.objExists(planeName + '*'):
cmds.delete(planeName + '*')
imgList = os.listdir(imgPlaneDir)
print(imgList)
selImg = imgList[value]
selImgPath = imgPlaneDir + '\{}'.format(selImg)
print(selImgPath)
os.chdir(imgPlaneDir)
cmds.imagePlane(c = selCam + 'Shape', n = planeName, fn = selImg)
cmds.setAttr(selCam + '_imgPlaneShape2.depth', 100)
window = cmds.window(title = 'Toggle_Image_Planes', widthHeight=(200, 55))
cmds.columnLayout( adjustableColumn = 1 )
selCtrl = cmds.intSliderGrp(min = 0, max = len(os.listdir(imgPlaneDir)) - 1, v = 0, s = 1, dc = 'empty')
cmds.intSliderGrp(selCtrl, e = 1, dc = partial(toggleImage, selCtrl))
cmds.setParent( '..' )
cmds.showWindow( window )
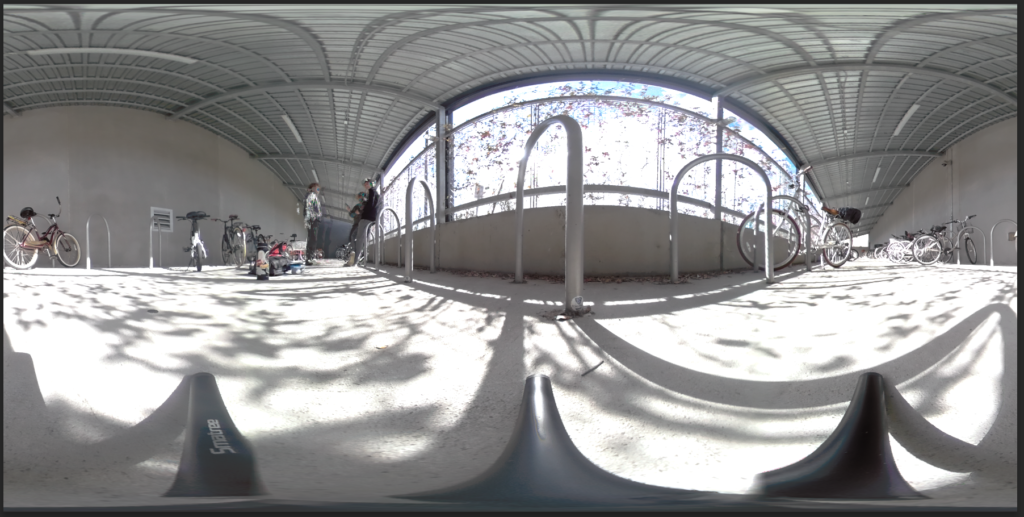